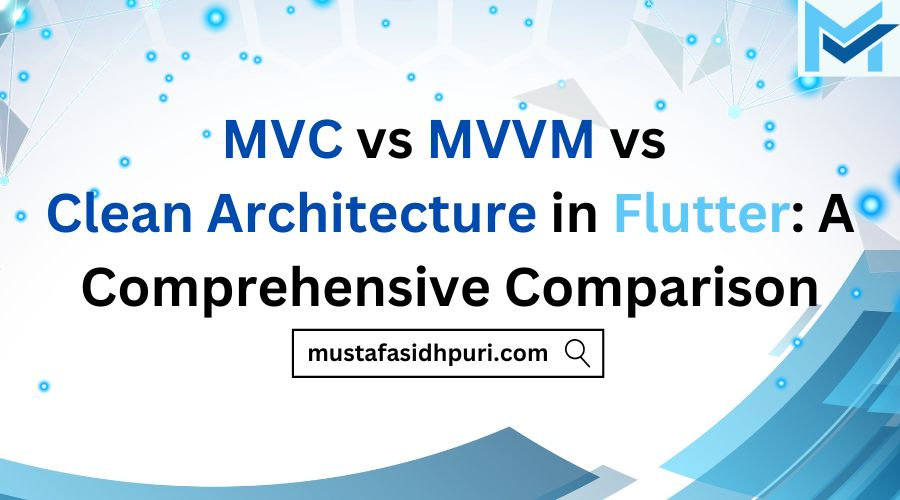
MVC vs MVVM vs Clean Architecture in Flutter: A Comprehensive Comparison
Oct 12, 2024
5 min read
0
91
0
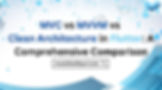
Introduction
When developing a Flutter application, choosing the right architecture is crucial for the code's maintainability, scalability, and testability. Three popular architectural patterns commonly discussed in Flutter development are Model-View-Controller (MVC), Model-View-ViewModel (MVVM), and Clean Architecture. Each pattern has its strengths and weaknesses and is suitable for different kinds of projects.
In this article, we will break down the key concepts of each architecture, provide code examples, highlight their pros and cons, and discuss common use cases. By the end, you will clearly understand which architecture suits your Flutter project best.
Table of Contents
1. What is MVC Architecture?
Overview of MVC in Flutter
The Model-View-Controller (MVC) is one of the oldest and most common design patterns used in software development. In this pattern, the application is divided into three primary components: Model, View, and Controller.
Model: The data layer, responsible for handling the business logic and managing the app’s data.
View: The UI layer, displaying data and sending user interactions to the Controller.
Controller: The intermediary between the Model and View. It listens to user inputs from the View, updates the Model, and then tells the View to update accordingly.
MVC Structure in Flutter
In Flutter, the View represents the widgets, the Model contains the app’s data, and the Controller manages the interaction between the two. Here’s a simplified representation:
Model <--> Controller <--> View
Example Code
Benefits of MVC
Separation of concerns: MVC separates the UI, logic, and data layers, making the application more structured.
Ease of use: Easy to understand for smaller applications and beginners.
Drawbacks of MVC
Tightly coupled components: In MVC, the View is tightly coupled with the Controller, which may lead to scalability issues as the project grows.
Difficult to scale: For larger applications, MVC can lead to convoluted code and decreased maintainability.
When to Use MVC
MVC is ideal for small to medium-sized projects or for teams that prefer a simple and easy-to-understand architecture. It’s a great choice for rapid prototyping or applications where the complexity is minimal.
2. What is MVVM Architecture?
Overview of MVVM in Flutter
The Model-View-ViewModel (MVVM) architecture is an evolution of MVC. In MVVM, the Controller is replaced by a ViewModel, which serves as an intermediary between the Model and the View, but with a few key differences. The ViewModel is responsible for exposing data to the View and handling UI-related logic.
Model: The data layer, similar to MVC.
View: The UI layer (Flutter widgets) that displays data.
ViewModel: Exposes data from the Model to the View and handles logic.
MVVM Structure in Flutter
In Flutter, the ViewModel typically holds the state of the UI and updates the View when necessary. Here’s a high-level structure:
Model <--> ViewModel <--> View
Example Code
Benefits of MVVM
Improved testability: Since the ViewModel is independent of the View, the application logic is easier to test.
Loose coupling: The ViewModel and View are loosely coupled, improving scalability.
Drawbacks of MVVM
Boilerplate code: MVVM can result in more code, especially for smaller applications.
Steep learning curve: The separation of View and ViewModel might confuse developers who are new to this pattern.
When to Use MVVM
MVVM is suitable for medium to large-scale projects that require scalability and testability. It’s a popular choice for applications with complex UI logic.
3. What is Clean Architecture?
Overview of Clean Architecture in Flutter
Clean Architecture, introduced by Robert C. Martin (Uncle Bob), focuses on creating systems that are easy to maintain, extend, and test. Clean Architecture splits the application into layers, with each layer depending only on the layer below it. In Flutter, this architecture is implemented using a series of domain, data, and presentation layers.
Clean Architecture Structure
Entities: Core business logic and entities.
Use Cases: Application-specific business rules that define how the app behaves.
Repositories: Data source handling, abstracted from the domain layer.
Presentation Layer: Flutter widgets (UI), interacting with ViewModels or Controllers.
Example Code
Benefits of Clean Architecture
Highly modular: Each layer is decoupled, making the codebase easier to extend and maintain.
Testability: With separate layers, unit tests can target specific parts of the app.
Scalable: Clean Architecture is ideal for complex applications with multiple data sources.
Drawbacks of Clean Architecture
Complexity: Clean Architecture can be overkill for small applications.
Initial setup: Setting up Clean Architecture involves a significant amount of boilerplate code.
When to Use Clean Architecture
Clean Architecture is best suited for large-scale applications with complex business logic and multiple layers of data interaction.
Comparing MVC, MVVM, and Clean Architecture
Architectural Layering
MVC: Three-layered approach (Model, View, Controller).
MVVM: Three-layered approach (Model, View, ViewModel) with loose coupling.
Clean Architecture: Multi-layered approach with a clear separation of concerns across business logic, data, and UI.
Ease of Implementation
MVC: Easiest to implement, especially for small projects.
MVVM: More challenging but offers better testability and scalability.
Clean Architecture: Most difficult to implement but provides the highest level of maintainability and scalability.
Maintainability and Scalability
MVC: Scales poorly as the application grows.
MVVM: Moderate scalability, better suited for medium projects.
Clean Architecture: Highly scalable and maintainable for large applications.
Testability
MVC: Difficult to unit test because of tight coupling between components.
MVVM: Highly testable due to separation of concerns.
Clean Architecture: The best in terms of testability since each layer can be tested independently.
Performance Considerations
MVC: May lead to performance bottlenecks in large applications.
MVVM: Offers better performance for complex UI interactions.
Clean Architecture: Can introduce overhead due to abstraction but ensures long-term maintainability.
Best Practices for Choosing the Right Architecture
For small projects with minimal complexity, choose MVC.
For medium-sized projects with moderate complexity and test requirements, use MVVM.
For large-scale applications with complex business logic and data handling, implement Clean Architecture.
Conclusion
Choosing the right architecture pattern for your Flutter project depends on the scale, complexity, and long-term goals of the application. While MVC is simple and easy to implement, it struggles with scalability. MVVM offers better testability and decoupling but can result in more boilerplate code. Clean Architecture, though complex, provides a highly modular and maintainable code structure ideal for large, scalable applications.
FAQs
What is the main difference between MVC and MVVM in Flutter?
MVC tightly couples the UI and logic, whereas MVVM decouples them, improving scalability and testability.
When should I use Clean Architecture in Flutter?
Clean Architecture is ideal for large-scale applications with complex business logic and multiple layers of data interaction.
Is MVVM better than MVC in Flutter?
MVVM is generally considered better for medium to large projects because it offers better testability and separation of concerns, but MVC is simpler for smaller apps.
What are the drawbacks of Clean Architecture?
The primary drawback of Clean Architecture is its complexity and the significant amount of boilerplate code required to set it up.
Can I switch from MVC to MVVM or Clean Architecture in Flutter?
Yes, it's possible, but it would require a significant refactor, especially in large projects. Start by decoupling the business logic and UI to ease the transition.